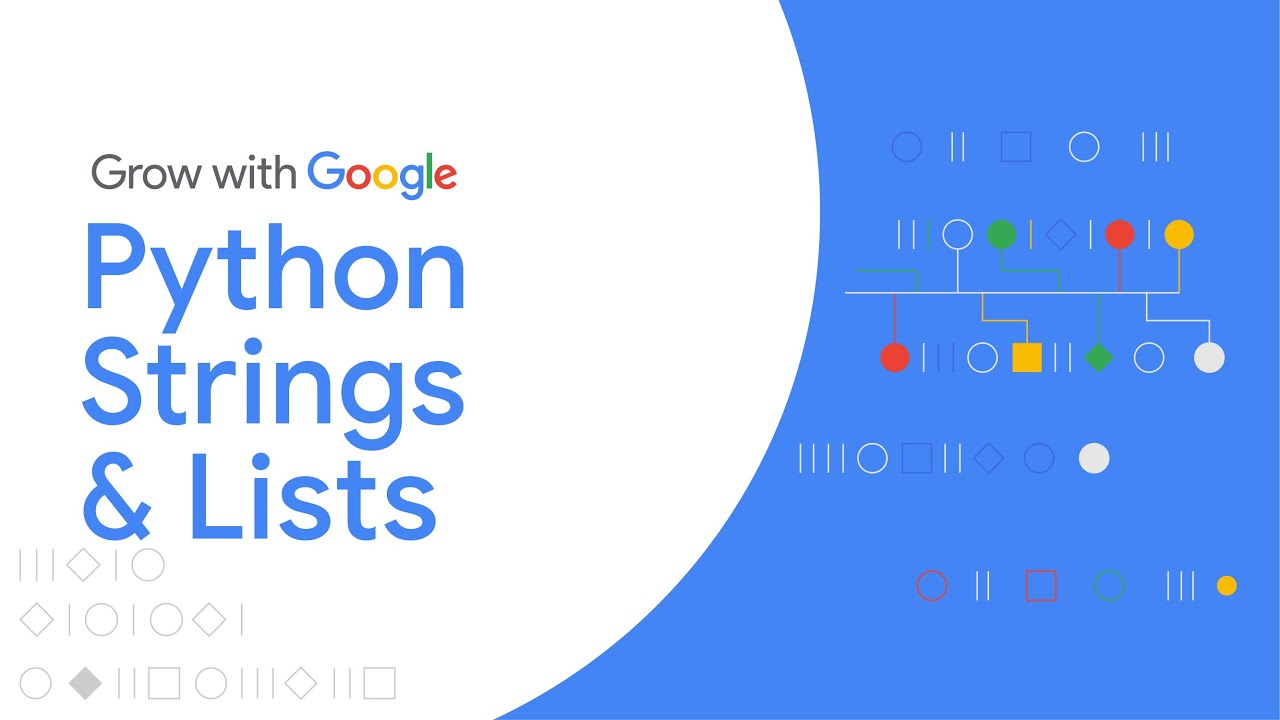
Mastering Python programming is essential for automation in IT careers. Strings, lists, and dictionaries are foundational data structures in Python that enhance how we control and manipulate data. This article dives deep into these data types, providing practical examples and insights into their use in automating tasks effectively.
Understanding Strings in Python
Strings in Python represent a sequence of characters enclosed within quotes. They can be created using either single or double quotes and are used for various purposes, such as holding text information like usernames, email addresses, and IDs.
Key Operations with Strings
- Concatenation: Combine strings using the
+
operator. For instance,"Hello, " + "World!"
results in"Hello, World!"
. - Repetition: Repeat strings with the
*
operator, e.g.,"ha" * 3
produces"hahaha"
. - Length: The built-in
len()
function determines the number of characters in a string.
Accessing Characters in Strings
Python allows you to access characters within a string using indexing. A zero-based index means:
- The first character is at index
0
- The last character can be accessed using negative indexing, e.g.,
string[-1]
gives you the last character.
You can also use slicing to get substrings. For example, string[start:end]
retrieves characters from the start
index up to, but not including, the end
index, allowing for flexible string manipulation.
Modifying Strings
One important point to note is that strings are immutable; they cannot be changed directly once created. Instead, modifications require creating a new string using methods or with concatenation techniques. For instance, to replace a term in a string, you could employ the replace()
method, which returns a new string:
new_string = old_string.replace("old", "new")
Common String Methods
Here are a few string methods handy for manipulating text:
str.lower()
: Converts all characters to lowercase.str.upper()
: Converts all characters to uppercase.str.strip()
: Removes whitespaces from both ends.str.split()
: Splits a string into a list based on space or specified character.
Working with Lists in Python
Lists are another vital data structure in Python, allowing you to maintain ordered collections of items. You can create a list by enclosing items within square brackets.
Creating and Accessing Lists
To create a list:
my_list = ["apple", "banana", "cherry"]
You can access list elements via indexing, similar to strings, and slices work in the same way as well.
Modifying Lists
Lists are mutable, meaning you can change their contents. Use the following methods to modify your lists:
- Appending:
append()
adds an item to the end of the list. - Inserting:
insert(index, value)
allows adding an item at a specified position. - Removing: Use
remove(value)
to delete the first occurrence orpop(index)
to remove an item at a specific index and return it.
Iterating Through Lists
You can utilize loops to iterate through lists:
for item in my_list:
print(item)
This method not only allows you to print each item but can also facilitate complex operations on list contents.
Delving Into Dictionaries in Python
Dictionaries are unordered collections of data that store items in key-value pairs, making them more accessible for searching than lists. The key serves as the identifier, and the value holds the data.
Creating and Accessing Dictionaries
Create a dictionary with curly brackets, specifying key-value pairs:
file_counts = {"jpg": 10, "txt": 14}
Access values using their key:
number_of_txt_files = file_counts["txt"]
Modifying Dictionaries
Dictionaries are also mutable, enabling you to add, remove, or change items:
- Adding a new key-value pair:
file_counts["csv"] = 5
- Updating an existing key:
file_counts["txt"] = 20
- Removing an entry:
del file_counts["jpg"]
Iterating Through Dictionaries
To loop through a dictionary, use for
loops and .items()
to get both keys and values:
for ext, num in file_counts.items():
print(f'There are {num} files with .{ext} extension')
When to Use Each Data Type
- Strings: Use them for managing textual data and information that is not meant to be modified.
- Lists: Best for storing ordered collections where the index is significant, such as user inputs, file names, or items in a shopping cart.
- Dictionaries: Ideal for scenarios where you need fast access to data via key-value pairs, such as configurations, lookups, or data aggregation.
Conclusion
Mastering strings, lists, and dictionaries is crucial for anyone looking to excel in Python programming, especially in IT automation tasks. Their flexibility and ease of use will significantly enhance your scripting capabilities.
Are you ready to elevate your Python skills? Start implementing these concepts in your projects and discover just how powerful Python can be!
For additional resources and detailed insights into Python programming and data manipulation, consider exploring DocsBot for content creation.
Happy coding!